Event System
Five uses publish subscriber mode to manage internal asynchronous behavior, you can use five.on()
or five.once()
to monitor asynchronous events such as Five life cycle and interactive feedback.Of course, you can also unsubscribe from callback functions via five.off()
.
Full Five Asynchronous events you can view from Five.EventCallback , This is only a brief description of commonly used asynchronous events.
Load Progress
From executing five.load(work)
to rendering the 3D panorama and model mainly goes through four key stages:
request cube panorama map ➩ request model map ➩ analyze 3D spatial data ➩ render 3D model
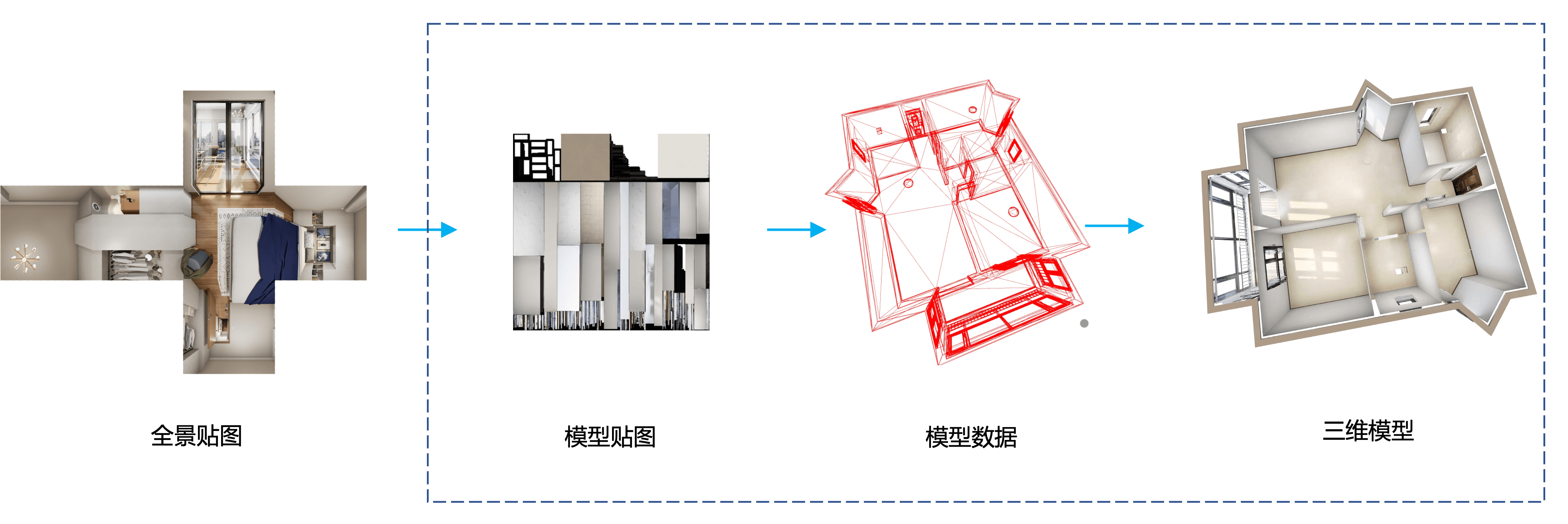
Panorama
In most scenarios, Five rendering default models is Panorama, allowing panorama to interact once six cube panorama maps are downloaded.
/* Your progress bar logic */
const progress = (pst: number) => {
if (pst === 1) {
five.off("textureLoading", progress);
}
const percentage = (pst * 0.75 + 0.2) * 100;
};
five.on("textureLoading", progress);
// The panorama has been rendered and can be interacted with at the current point
five.once("panoArrived", () => {});
::caution
five.on('textureLoading')
only indicates download progress of the panoramic picture, with five.once('panoArrived')
There may be a delay of about 100 ms with full scope interaction.
:::
Model Status
five.on("modelMaterialLoaded", () => {
/* model material loaded */
});
five.on("modelGeometryLoaded", () => {
/* model triangle data loaded */
});
five.on("modelLoaded", () => {
/* model loaded */
});
Status Change
As mentioned earlier, the Five state can be obtained and modified through the five.setState()
and five.state()
methods.
You can get five.on('stateChange')
for Five status changes:
five.on("stateChange", (newState, oldState, userAction) => {});
userAction
indicates whether changes are made by users by clicking on, rotation, etc.
Panorama Walking
InPanorama
panorama walk mode , you can walk in space (switch panorama position), there will also be some key events here:
five.on("wantsMoveToPano", (panoIndex) => {
/* Intention to a certain point */
return false; /* If the return value is false, the subsequent behavior will be aborted */
});
five.on("panoWillArrive", (panoIndex) => {
/* ready to go to a certain point */
});
five.on("movingToPano", (panoIndex) => {
/* going to a certain point Bit moving process */
});
five.on("panoArrived", (panoIndex) => {
/* has reached a certain point */
});
Mode switch
In addition to walking between points in the panoramic mode, there are also related events when switching between:.
five.on("wantsChangeMode", (mode: Mode, prevMode: Mode) => {
/* will switch mode:
can be blocked by returning false */
});
five.on("modeChange", (mode: Mode, prevMode: Mode) => {
/* Mode, but the UI effects are not necessarily fully rendered in the new model, with an animation*/
});
You need to note that the inter-mode switch is attached and can listen to the animation execution event:
five.on("initAnimationWillStart", () => {
/* Models toggle start */
});
five.on("initAnimationEnded", () => {
/* Models tow end */
});
Gesture action
// Gesture action
five.on(
"gesture",
(
// GestureType: "pan" | "tap" | "pinch" | "press" | "mouseWheel"
type: GestureTypes,
// Multiple finger
pointers: { delta?: number; x: number; y: number }[],
// end of
final: boolean
) => {}
);
Slide Screen
five.on("wantsPanGesture", (pose: Pose, final: boolean) => {
// Swipe the screen gesture is about to be triggered (not yet fired)
// can be stopped by returning false for
});
five.n("panGesture", (pose: Pose, final: boolean) => {
// Slide screen gestures triggered
});
Click on the screen
// Below 250 ms
five.on(
"wantsTapGesture",
(
raycaster: Raycaster,
tapPosition: Vector2,
duration: number,
final: boolean
) => {
/// Touch the screen gesture to be triggered (not yet fired)
// can be stopped by returning false to prevent the trigger
}
);
five.on(
"tapGesture",
(
raycaster: Raycaster,
tapPosition: Vector2,
duration: number,
final: boolean
) => {
// Touch screen gesture to be triggered
}
);
// More than 250 ms
five.on(
"wantsPressGesture",
(
raycaster: Raycaster,
tapPosition: Vector2,
duration: number,
final: boolean
) => {
// Clicking the screen gesture will be triggered (not yet fired)
// can be stopped by returning false to prevent trigger
}
);
five.on(
"pressGesture",
(
raycaster: Raycaster,
tapPosition: Vector2,
duration: number,
final: boolean
) => {
// Click on the screen to be triggered
}
);
Two finger zoom
five.on("wantsPinchGesture", (pose: Pose, final: boolean) => {
// Two finger zoom gesture will be triggered (not yet fired)
// can be stopped by returning false for
});
five.on("pinchGesture", (pose: Pose, final: boolean) => {
// Two finger zoom gestures will be triggered (not yet fired)
// Can be prevented from triggering by returning false
});
Mouse Wheel
five.on("wantsMouseWheel", (delta: number, fov: number, final: boolean) => {
// mouse wheel gesture will be triggered (not triggered yet)
// trigger can be prevented by return false
});
five.on("mouseWheel", (delta: number, fov: number, final: boolean) => {
// mouse wheel gesture is triggered
});
Focus Circle
There is a focus circle inside the three-dimensional space, leading to the current mouse or touch screen area. The position and direction of the focus circle will be recalculated when moving gestures.
five.on(
"intersectionOnModelUpdate",
(
intersection: Intersection, // focus collision detection result
mesh: IntersectMeshInterface // focus ring
) => {
// mouse focus ring position is recalculated
}
);